Dev C++ Does Not Display Output
Originally released by Bloodshed Software, but abandoned in 2006, it has recently been forked by Orwell, including a choice of more recent compilers. It can be downloaded from:
http://orwelldevcpp.blogspot.com
Installation
Run the downloaded executable file, and follow its instructions. The default options are fine.Support for C++11
By default, support for the most recent version of C++ is not enabled. It shall be explicitly enabled by going to:Tools -> Compiler Options
Here, select the 'Settings' tab, and within it, the 'Code Generation' tab. There, in 'Language standard (-std)' select 'ISO C++ 11':
Ok that. You are now ready to compile C++11!
Compiling console applications
To compile and run simple console applications such as those used as examples in these tutorials it is enough with opening the file with Dev-C++ and hitF11
.As an example, try:
File -> New -> Source File
(or Ctrl+N
)There, write the following:
Then:
File -> Save As..
(or Ctrl+Alt+S
)And save it with some file name with a
.cpp
extension, such as example.cpp
.Now, hitting
F11
should compile and run the program.If you get an error on the type of
x
, the compiler does not understand the new meaning given to auto
since C++11. Please, make sure you downloaded the latest version as linked above, and that you enabled the compiler options to compile C++11 as described above.Tutorial
You are now ready to begin the language tutorial: click here!.- Dev C++ Is Not Showing Output
- Dev C++ Does Not Display Output Work
- Dev C++ Does Not Display Output Windows 10
1) After compilation and execution using ctrl + F9, go to menu window-output. You'll see the latest output window. 2) Just add getch or getche function at the end of your main. It will hold the window for user input ( Specifically waits fo. Jan 08, 2018 How to Reset Dev C default Settings. Restore default settings in Dev C. Dev C plus plus is an IDE for writing programs in C and C. Learn how to reset default settings in Dev C. Nov 08, 2014 Actually this problem is solved in the newer version of the Dev C.So either download that. Or Just put a getchar at the end of your code just before the return statement and so after the code completes its execution, the terminal window would. Dev-C is a free IDE for Windows that uses either MinGW or TDM-GCC as underlying compiler. Originally released by Bloodshed Software, but abandoned in 2006, it has recently been forked by Orwell, including a choice of more recent compilers.
Redirection
One way to get input into a program or to display output from a program is to use standard input and standard output, respectively. All that means is that to read in data, we use cin
(or a few other functions) and to write out data, we use cout
.
When we need to take input from a file (instead of having the user type data at the keyboard) we can use input redirection:
This allows us to use the same cin
calls we use to read from the keyboard. With input redirection, the operating system causes input to come from the file (e.g., inputfile
above) instead of the keyboard.
Similarly, there is output redirection:
Dev C++ Is Not Showing Output
that allows us to use cout
as before, but that causes the output of the program to go to a file (e.g., outputfile
above) instead of the screen.
Of course, the 2 types of redirection can be used at the same time..
C++ File I/O
While redirection is very useful, it is really part of the operating system (not C++). In fact, C++ has a general mechanism for reading and writing files, which is more flexible than redirection alone.
iostream.h and fstream.h
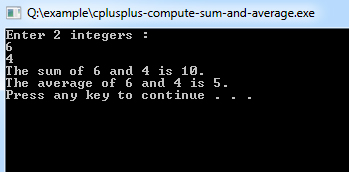
There are types and functions in the library iostream.h that are used for standard I/O. fstream.h includes the definitions for stream classes ifstream (for input from a file), ofstream(for output to a file) and fstream (for input to and output from a file). Make sure you always include that header when you use files.
Dev C++ Does Not Display Output Work
Type
For files you want to read or write, you need a file stream object, e.g.:
Functions
Reading from or writing to a file in C++ requires 3 basic steps:
- Open the file.
- Do all the reading or writing.
- Close the file.
Following are described the functions needed to accomplish each step.
- Opening a file:
In order to open a file, use the member function
open()
. Use it as:where:
- filename is a string that holds the name of the file on disk (including a path like
/cs/course
if necessary). - mode is a string representing how you want to open the file. Most often you'll open a file for reading (
ios::in
) or writing (ios::out or ios::app
).
Note that
open()
initializes the file object that can thenbe used to access the file. After opening the file, we should test thefile object (e.g., with!
below) to make sure it wasproperly opened (e.g., an open may fail if we don't have the correctpermissions or the file doesn't exist when opening for reading).Auto tune recorder online free. Auto-tune Pro 9.1 overviewAuto-Tune Pro is the most complete and advanced edition of Auto-Tune. It is full offline installer standalone setup of Auto-tune Pro 9.1 free download with the crack download for x32/x64 Bit version.
Here are examples of opening files:
Note that the input file that we are opening for reading (
Note: There are other modes you can use when opening a file,such as append (ios::in
) must already exist. In contrast, the output file we are opening for writing (ios::out
) does not have to exist. If it does not, it will be created. If this output file does already exist, its previous contents will be thrown away (and will be lost).ios::app
) to append something to the end ofa file without losing its contents..or modes that allow you to bothread and write. - filename is a string that holds the name of the file on disk (including a path like
- Reading from or writing to a file:
Once a file has been successfully opened, you can read from it in the same way as you would read with
cin
or write to it in the same way as you write usingcout
.Continuing our example from above, suppose the input file consists of lines with a username and an integer test score, e.g.:
and that each username is no more than 8 characters long.
We might use the files we opened above by copying each username and score from the input file to the output file. In the process, we'll increase each score by
10 points for the output file:In the
while
loop, we keep on readingusername
andscore
until we hit the end of thefile. This is tested by calling the member functioneof()
.The bad thing about using
eof()
is that if the file is notin the right format (e.g., a letter is found when a number isexpected):then
>>
will not be able to read that line (since there is no integer to read) and it won't advance to the next line in the file. For this error,eof()
will not return true (it's not at the end of the file)..Errors like that will at least mess up how the rest of the file is read. In some cases, they will cause an infinite loop.
One solution is to test against the number of values we expect to beread by
>>
operator each time. Since there are twotypes a string and an integer, we expect it to readin 2 values, so our condition could be:Sep 06, 2018 i love this autotune thing, Reply. 64-bit 2018 2019 analog au bass best DAW delay Download easy Editor edm eq fm free free download Full fx help high sierra hip hop izotope MAC mastering microsoft mixing mojave native instruments os x osx plugin Plugins release reverb sine sound design studio synth synthesizer techno trance vst windows. Antares autotune vst free crack download windows.
Now, if we get 2 values, the loop continues. If we don't get 2 values,either because we are at the end of the file or some other problemoccurred (e.g., it sees a letter when it is trying to read in anumber), then the loop will end (
Note: When you use>>
will return a 0in this case).eof()
, it will not detect the end of the file until it tries to read past it. In other words, they won't report end-of-file on the last valid read, only on the one after it. - Closing a file:
When done with a file, it must be closed using the member function
close()
.To finish our example, we'd want to close our input and output files:
Closing a file is very important, especially with output files. The reason is that output is often buffered. This means that when you tell C++ to write something out, e.g.,
it doesn't necessary get written to disk right away, but may end up in a buffer in memory. This output buffer would hold the text temporarily:
(The buffer is really just 1-dimensional despite this drawing.)
When the buffer fills up (or when the file is closed), the data is finally written to disk.
So, if you forget to close an output file then whatever is still in the buffer may not be written out.
Note: There are other kinds of buffering than the one we describe here.
A complete program that includes the code above, plus input files touse with that program, is available to download.
Special Files
There are 3 special file objects that are always defined for a program. They are cin
(standard input), cout
(standard output) and cerr
(standard error).
Standard Input
Standard input is where things come from when you use cin
. For example,
Standard Output
Similarly, standard output is exactly where things go when you use cout. For example,
Remember that standard input is normally associated with the keyboard and standard output with the screen, unless redirection is used.
Dev C++ Does Not Display Output Windows 10
Standard Error
Standard error is where you should display error messages. We've already done that above:
Standard error is normally associated with the same place as standard output; however, redirecting standard output does not redirect standard error.
For example,
only redirects stuff going to standard output to the file outfile.. anything written to standard error goes to the screen.
BU CAS CS - Intro to File Input/Output in C++This page created by Saratendu Sethi <sethi@cs.bu.edu>.
Material adapted for C++ from Intro to FileInput/Output in C.